桥接模式是一种结构型设计模式,将抽象和实现解耦,让它们可以独立变化
逻辑结构
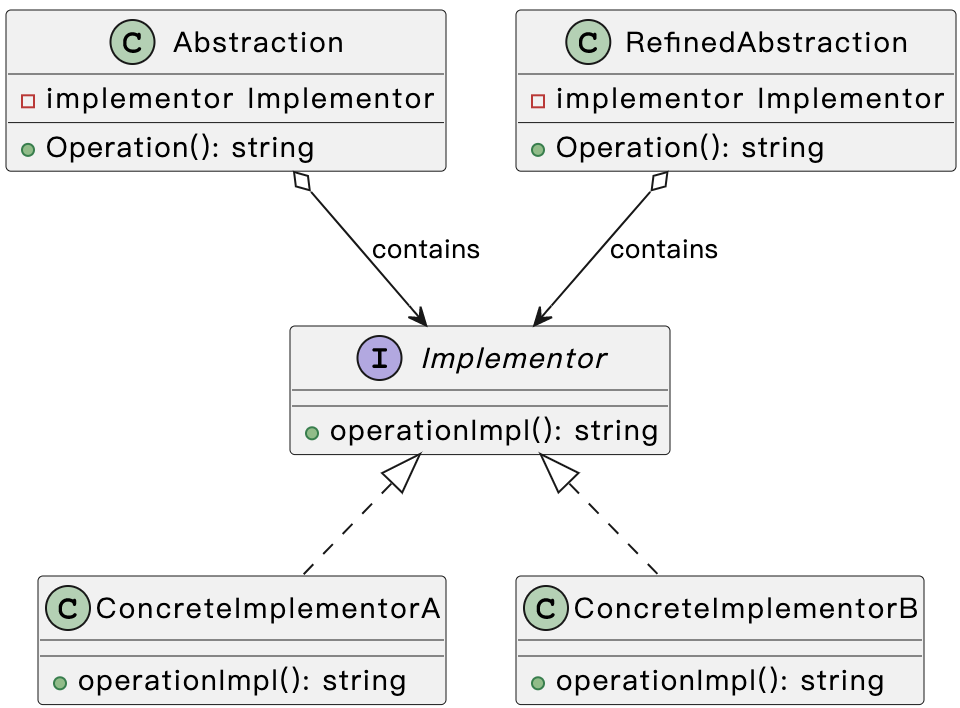
代码实现
代码路径:https://github.com/XBoom/DesignPatterns/tree/main/08_Bridge
-
定义实现类的接口
1
2
3type Implementor interface {
operationImpl() string
} -
实现具体的实现类
1
2
3
4
5
6
7
8
9
10
11type ConcreteImplementorA struct{}
func (c *ConcreteImplementorA) operationImpl() string {
return "ConcreteImplementorA"
}
type ConcreteImplementorB struct{}
func (c *ConcreteImplementorB) operationImpl() string {
return "ConcreteImplementorB"
} -
定义抽象类及其方法
1
2
3
4
5
6
7type Abstraction struct {
implementor Implementor
}
func (a *Abstraction) Operation() string {
return a.implementor.operationImpl()
} -
实现具体的抽象类
1
2
3
4
5
6
7type RefinedAbstraction struct {
Abstraction
}
func NewRefinedAbstraction(implementor Implementor) *RefinedAbstraction {
return &RefinedAbstraction{Abstraction{implementor}}
}
通过以上步骤,就可以使用桥接模式了。例如使用 ConcreteImplementorA
类来完成某个操作
1 | implementorA := &ConcreteImplementorA{} |
适用场景
- 想要拆分或重组一个具有多重功能的庞杂类(例如能与多个数据库服务器进行交互的类),可以使用桥接模式
- 希望在几个独立维度上扩展一个类
- 需要在运行时切换不同实现方法
总结
- 开闭原则。 你可以新增抽象部分和实现部分, 且它们之间不会相互影响。
- 单一职责原则。 抽象部分专注于处理高层逻辑, 实现部分处理平台细节
- 桥接模式需要额外的工作量来设计抽象和实现部分的接口,并且增加了系统的理解难度。
- 如果某个类在多个维度上都有变化,那么桥接模式可能会导致类的数量急剧增加,从而增加系统的复杂度