迭代器模式是一种行为设计模式, 让你能在不暴露集合底层表现形式(列表、 栈和树等) 的情况下遍历集合中所有的元素
逻辑结构
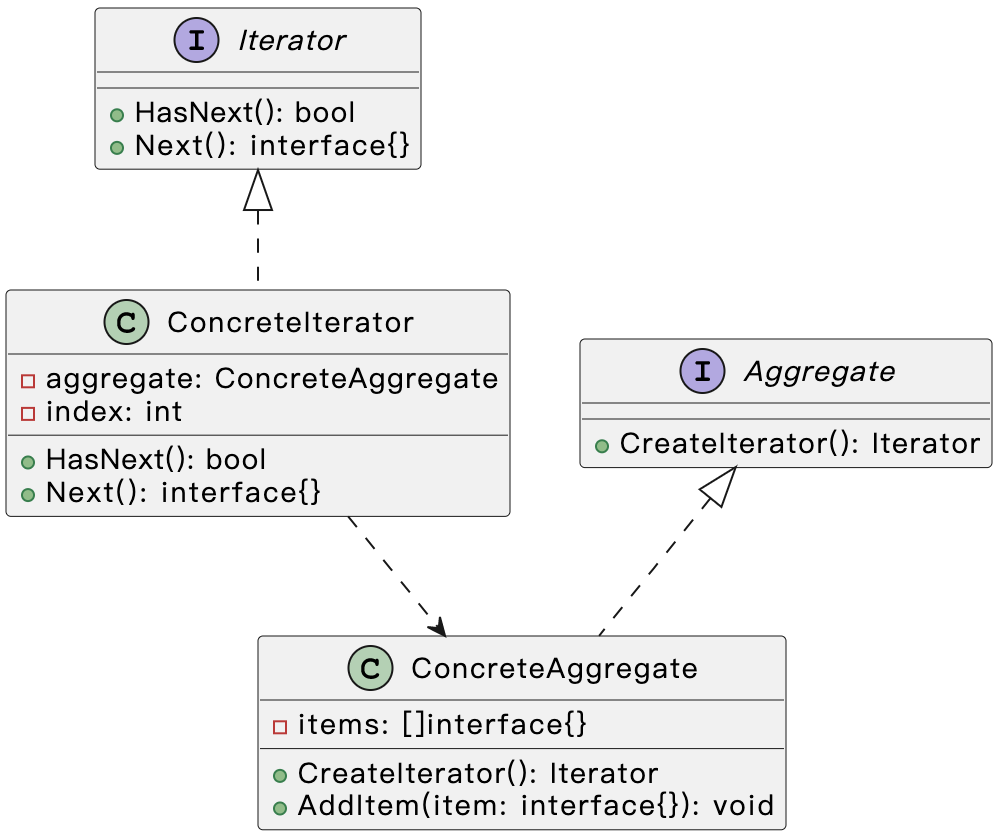
代码实现
代码路径:https://github.com/XBoom/DesignPatterns/tree/main/19_Iterator
-
定义一个迭代器接口
Iterator
,该接口包含了访问聚合对象元素的方法1
2
3
4
5// Iterator 迭代器接口
type Iterator interface {
HasNext() bool
Next() interface{}
} -
定义一个聚合对象接口
Aggregate
,该接口包含了创建迭代器对象的方法1
2
3
4// Aggregate 聚合对象接口
type Aggregate interface {
CreateIterator() Iterator
} -
接着,实现具体的迭代器结构体
ConcreteIterator
,它实现了Iterator
接口:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15// ConcreteIterator 具体迭代器
type ConcreteIterator struct {
aggregate *ConcreteAggregate
index int
}
func (i *ConcreteIterator) HasNext() bool {
return i.index < len(i.aggregate.items)
}
func (i *ConcreteIterator) Next() interface{} {
item := i.aggregate.items[i.index]
i.index++
return item
} -
再实现具体的聚合对象结构体
ConcreteAggregate
,它实现了Aggregate
接口,并在CreateIterator
方法中返回具体的迭代器对象1
2
3
4
5
6
7
8
9
10
11
12
13
14
15// ConcreteAggregate 具体聚合对象
type ConcreteAggregate struct {
items []interface{}
}
func (a *ConcreteAggregate) CreateIterator() Iterator {
return &ConcreteIterator{
aggregate: a,
index: 0,
}
}
func (a *ConcreteAggregate) AddItem(item interface{}) {
a.items = append(a.items, item)
}
运行
1 | aggregate := &ConcreteAggregate{} |
结果
1 | Item 1 |
适用场景
- 集合类容器的遍历:使用迭代器模式可以方便地遍历集合类容器(如数组、链表、哈希表等)中的元素,而无需关心容器内部的具体实现方式。
- 文件系统遍历:对于文件系统中的目录和文件,可以使用迭代器模式进行遍历操作,递归地访问每个目录并获取其中的文件信息。
- 数据库结果集遍历:在数据库操作中,可以使用迭代器模式遍历查询结果集,逐行获取数据并进行相应的处理。
总结
- 单一职责原则。 通过将体积庞大的遍历算法代码抽取为独立的类, 对客户端代码和集合进行整理。
- 开闭原则。 可实现新型的集合和迭代器并将其传递给现有代码, 无需修改现有代码。
- 可以并行遍历同一集合, 因为每个迭代器对象都包含其自身的遍历状态。
- 相似的, 可以暂停遍历并在需要时继续。